
Are you tired of tripping over complicated code in your JavaScript adventures? Async functions are like supercharged sidekicks, letting your programs multitask without breaking a sweat.
In this blog post, we'll uncover the secrets to mastering these async allies, making your coding life easier and more efficient. Get ready for a fun ride into the world of async magic!
Understanding Async Functions in JavaScript
Async functions in JavaScript allow for non-blocking, asynchronous code execution. They utilize the 'promise' and 'await' keywords to manage asynchronous operations effectively.
Definition
Async functions are a cool part of JavaScript programming. They let you write code that doesn't stop or block when it's waiting for something like loading data. Think of it like sending your friend to buy snacks and still being able to watch TV while they're out.
When they come back, you can enjoy the snacks! That's how async functions work with tasks in code.
They return a Promise, which is an object that represents work that will finish later. You use the await keyword before calling an async function. This tells JavaScript to wait for the Promise to do its thing before moving on with other tasks in your program.
It keeps your app running smoothly without delays or freezing up while waiting for data or actions to complete.
Syntax
Async functions in JavaScript are defined using the "async" keyword before the function declaration. This syntactic feature allows the function to operate asynchronously, meaning that it won't block other code from executing while waiting for an asynchronous operation to complete.
To work with async functions, you use the "await" keyword within the function body to indicate where the execution should pause and wait for a promise to resolve before moving on to the next line of code.
When using parameters with async functions, they behave just like regular synchronous functions, making them flexible for various programming needs.
Parameters
When defining async functions in JavaScript, the "async" keyword is used before a function declaration to turn it into an asynchronous function. This allows the function to operate asynchronously by using the "await" keyword inside its body.
The await keyword can only be used within async functions and provides a non-blocking way to pause execution until a promise is settled, returning its result.
Parameters of async functions can include any type of value, whether they are primitive values or objects. When calling an async function, it returns a promise that resolves with the value returned by the function upon completion or rejects with an uncaught exception thrown from within the function.
Execution order
Async functions are executed in a non-blocking manner, allowing other code to run without waiting. The execution order of async functions is important for understanding their behavior:
- Asynchronous code inside async functions is started but does not block the rest of the code.
- The function execution continues with the next line while waiting for the asynchronous operation to complete.
- Once the asynchronous operation finishes, the callback function is placed in the event queue.
- When the event loop selects the callback function, it's executed, and its result affects the further program flow.
- This allows multiple asynchronous operations to be initiated and then handled in a specific order, providing flexibility in managing concurrent tasks.
Benefits and Criticisms of Async Functions
Improved readability, easier error handling, and potential for callback hell are all important aspects to consider when working with async functions in JavaScript. Read on to discover how these elements can impact your programming experience!
Improved readability
Using async functions in JavaScript can significantly enhance the readability of code. By allowing asynchronous operations to be written in a synchronous style, it becomes easier for developers to understand and maintain the codebase.
This leads to clearer and more organized code, ultimately making it more accessible for other team members to work with.
By using async functions, the flow of the program becomes more intuitive as compared to traditional callback-based approaches. It simplifies error handling and makes it easier to follow the sequence of operations, contributing to improved overall readability and comprehension of the codebase.
Easier error handling
Async functions in JavaScript make error handling easier by allowing developers to use try-catch blocks. When an error occurs within an async function, it can be caught and handled gracefully using familiar error-handling techniques.
This simplifies the process of dealing with errors that may arise during asynchronous operations, creating more robust and reliable code. Additionally, async/await syntax makes it easier to write asynchronous code that is more readable and maintainable than traditional callback-based approaches.
Understanding how async functions streamline error handling is crucial for writing stable and efficient JavaScript applications. With advancements in asynchronous programming, developers can leverage these features to create smoother user experiences by managing errors effectively within their codebase.
Potential for callback hell
Async functions in JavaScript can lead to callback hell, a situation where nested callbacks become difficult to manage and understand. When multiple asynchronous operations are involved, the code structure can become complex and challenging to maintain.
This complexity arises from deeply nested functions, making it harder to trace the flow of the program. It also increases the likelihood of errors and reduces readability.
To mitigate callback hell, developers often turn to solutions such as promises or async/await syntax. By utilizing these alternatives, code becomes more linear and easier to comprehend, enhancing the overall quality of asynchronous programming within JavaScript.
Implementations of Async Functions
Discover how async functions are implemented in various programming languages and explore real-world examples of their usage in modern web development.
In various programming languages
Async functions are used not only in JavaScript but also in other programming languages. Let's explore how async functions are implemented in different programming languages:
- Python: Async functions can be created using the `async` and `await` keywords, allowing for non-blocking operations within Python programs.
- C#: In C#, async/await keywords enable developers to write asynchronous code that runs concurrently, improving responsiveness.
- Java: With the introduction of CompletableFuture, Java now supports async functions, enhancing its capabilities for handling asynchronous tasks.
- Kotlin: Kotlin provides support for coroutines, which allows developers to write asynchronous code more effectively and legibly.
- Rust: In Rust, async/await functionality is available through libraries like Tokio, enabling efficient concurrent operations without sacrificing performance.
Real-world examples
Async functions are commonly used in various real-world scenarios. They can be seen in web development for handling user input and fetching data from APIs. Additionally, they are utilized in creating responsive interfaces and managing multiple tasks concurrently.
- Online shopping platforms use async functions to update product availability without disrupting the user experience.
- Social media sites employ async functions to load new content dynamically as users scroll through their feeds.
- Gaming applications utilize async functions to manage user interactions, such as player movements and game state updates.
- Financial services rely on async functions for processing transactions and retrieving account information securely.
- Streaming platforms use async functions to deliver seamless video playback while simultaneously fetching recommended content based on user preferences.
How Async Functions Can Enhance Programming
By exploring the power of async functions, programmers can create generative art and unique experiences for collectors using non-fungible tokens (NFTs). This also opens up opportunities for collaboration and community involvement in the programming world.
Creating generative art
Generative art, powered by async functions in JavaScript, opens doors to unique and dynamic visual experiences. With non-blocking capabilities, code can generate endless variations of artwork using algorithms and randomness.
This allows for the creation of NFTs (Non-Fungible Tokens) that offer one-of-a-kind digital pieces to collectors, providing an innovative blend of technology and creativity.
By leveraging async functions in JavaScript programming, artists can collaborate with developers to produce generative art that responds to user input or environmental data. This fosters a vibrant community where individuals can actively participate in the art-making process through interactive installations or online platforms.
Unique experiences for collectors
Collectors can use async functions in JavaScript to create one-of-a-kind non-fungible tokens (NFTs) with event-driven programming. By leveraging nonblocking functions, JavaScript frameworks, and libraries, collectors can unleash their creativity and develop unique digital art pieces that are tokenized on the blockchain.
This enables them to offer exclusive digital collectibles which are rare and highly sought after in the NFT marketplace.
Async functions also allow collectors to collaborate with artists and programmers from around the world to bring innovative ideas to life. They can actively contribute to the development of generative art using callback functions, creating a new level of engagement within the community.
Collaboration and community involvement
Async functions in JavaScript open up opportunities for collaboration and community involvement by simplifying the development process. They allow developers to work together more effectively, sharing code and building on each other's contributions.
Through collaboration, innovative NFTs (non-fungible tokens) can be created with unique experiences for collectors, leveraging event-driven programming and JavaScript libraries to bring generative art to life.
Moreover, these async functions create a supportive environment where programmers can share their knowledge and skills, leading to the growth of open-source communities focused on creating new possibilities within the NFT space.
Conclusion
In conclusion, async functions in JavaScript offer improved readability and easier error handling. They provide practical solutions for event-driven programming needs and hold great potential for creating NFTs.
Emphasizing their impact can lead to significant improvements in coding efficiency and user experience. Have you considered implementing async functions in your next programming project? Explore further resources to enhance your understanding of this powerful JavaScript feature.
RelatedRelated articles
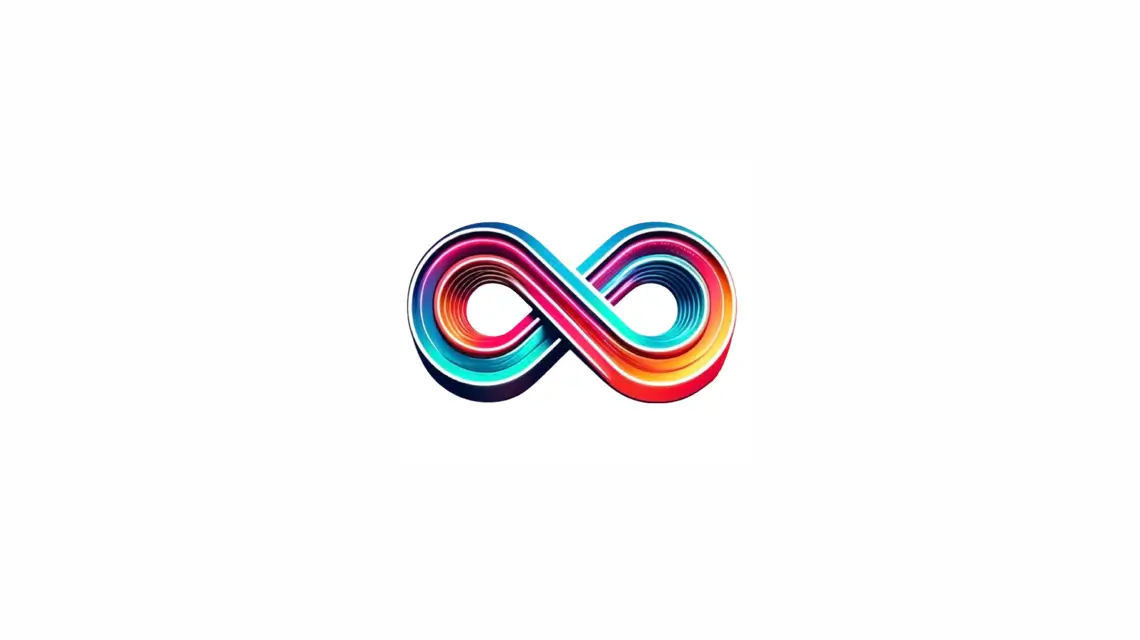
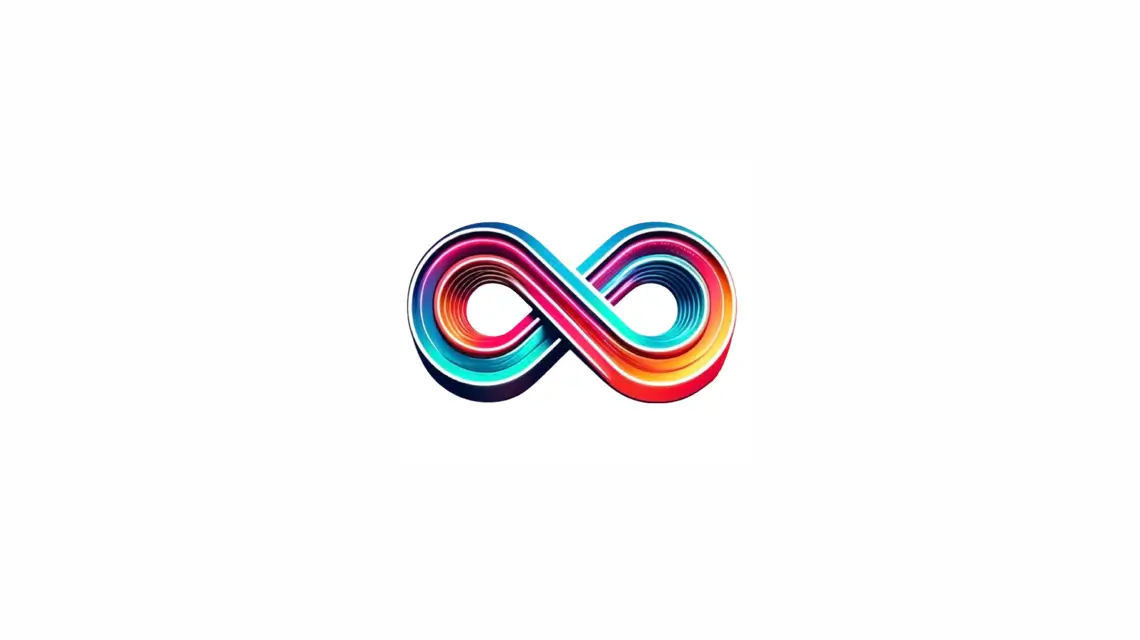
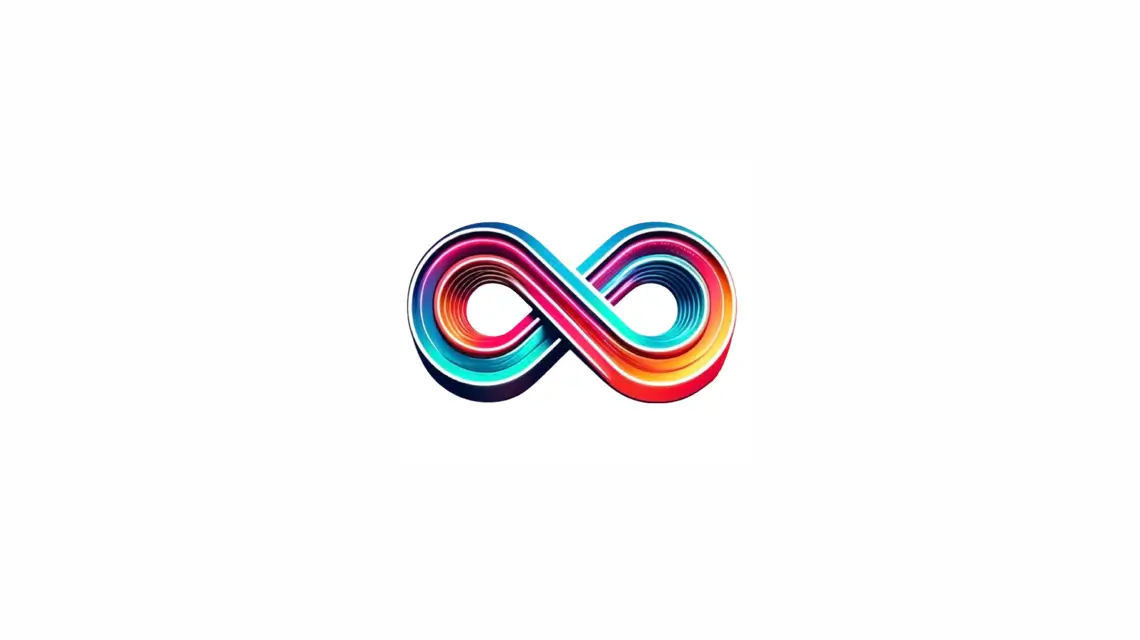